Object-Oriented Programming Core Concepts Part 2
- Rosmina Joy Cabauatan
- Apr 7, 2021
- 4 min read
Updated: Apr 8, 2021
What is an interface?
An object has an interface as its outward appearance. It is how other objects see the object. It is also considered as the attributes and methods that the object exposes. Usually, an object will only expose some of its attributes and methods. The object itself uses some attributes and methods. Therefore, no other object should have access to those attributes and methods. Some attributes and methods may be made accessible only to certain other objects and may be available in any other object.
The figure below shows shape as an interface. The attributes of the interface, like area, can be implemented in a circle, triangle, and square. However, the circle has its properties like radius, the triangle has the base and height and the square has the sides. The attributes are distinct for each shape, but all the shapes share the same attribute area.

Three Principles of Object-oriented Programming
Encapsulation
Inheritance
Polymorphism
Encapsulation is a mechanism of grouping interrelated attributes or variables and methods or operations as one unit. However, unrelated data and operations should not be separated from other units. Encapsulated attributes of a class can be hidden from other classes and can be accessed only by the functions of the active class. This is also referred to as data hiding. The figure below shows a class with encapsulated attributes, constructors, and methods as its properties.

To achieve encapsulation in Java,
Declare the attributes of a class as private, and
Provide public setter and getter methods to modify and view the values of the variables.
Encapsulation provides the following benefits:
The attributes of a class can be made read-only or write-only
A class can have total control over what is stored in its attributes
Simpler interfaces
Only a few methods are exposed to other objects.
Data are protected from corruption
Easier to modify code or find bugs
Simpler interactions
Examples of encapsulation are as follows:
Purchase Order Object
Data for purchase orders should not be lumped in the same objects as data for invoices and receipts
The methods for retrieving data from a purchase order should not be found in a separate class from the data.
DB Connection Object
The DB Connection object should not need to look up the URL from the database from another object every time it does an operation.
Information Hiding
An object should only expose what is necessary, and only at the appropriate level.
Car
Driver: Only the steering wheel, pedals, and stick shift are exposed. The driver should not access entire or gears or axle to drive the car
Mechanic
Access to the engine, gears, etc., but not the internals of each part
Manufacturer
Access to the internals of each part
The second principle of object-oriented programming is inheritance.
Inheritance is a mechanism for creating a new class by deriving from another class. It is the mechanism in Java by which one class is allowed to inherit the features(fields and methods) of another class.
There are two categories of applying inheritance in Java programming. The first is interface inheritance. In this category, the new class acquires the interface of the old class. The second is implementation inheritance. The new class in this category often acquires the implementation of the old class. A new class can change the implementation of the more former class or add its methods and attributes.
Implementation inheritance can be further classified as follows. The figure below shows the single, multilevel, and hierarchical classifications. In the single classification, SubClass inherits the properties of SuperClass. In this case, SuperClass is the parent class while SubClass is the child class. In the multilevel classification,SuperClass1 is the parent class and SubClass1 is the child class. However, since SubClass2 inherits the properties of SubClass1, SubClass1 becomes a parent class of the class. Hence, in this case, there are two levels of inheritance. In the hierarchical classification, there are two child classes namely, SubClass1 and SubClass2. These two classes inherit the properties of parent class SuperClass.

The figure below shows other classifications of inheritance. In contrast to the multilevel classification, the multilevel classification has two parent classes, SuerClass1 and SuperClass2 that are inherited by SubClass. In the hybrid classification, SuperClass serves as the parent class that is inherited by Sub/SuperClass1 and Sub/SuperClass2. In this case, Sub/SuperClass1 and Sub/SuperClass2 are child classes. However, since SubClass3 inherits the properties of Sub/SuperClass1 and Sub/SuperClass2, they can also serve as parent classes.

Inheritance is known as is-a type of relationship. The figure below shows an example. The TeachingEmployee and Non-Teaching Employee are child classes and the University Employee is the parent class. The relationship between the classes is called the IS-A relationship. It means that both Teaching and Non-Teaching employees are types of University Employees.
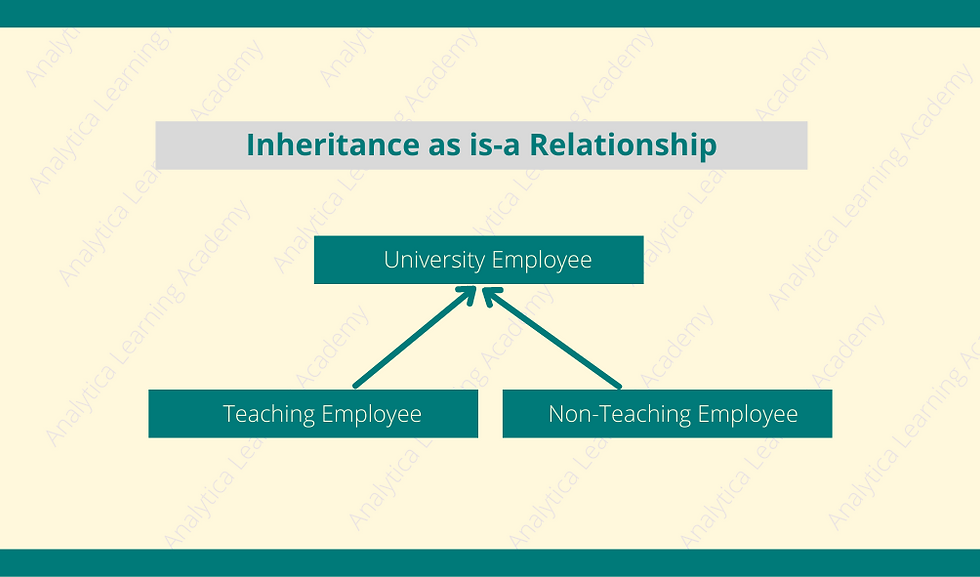
The last principle of object-oriented programming is polymorphism.
Polymorphism happens when a single data type exhibits different behaviors during execution. In Greek, Poly means "many", and morph means "form" so, polymorphism means "existing in many forms". The figure below shows an example of the polymorphic characteristics of the Shape class. The class Shape has three forms as Circle, Triangle, and Rectangle. These forms have different ways of implementing the area attribute.
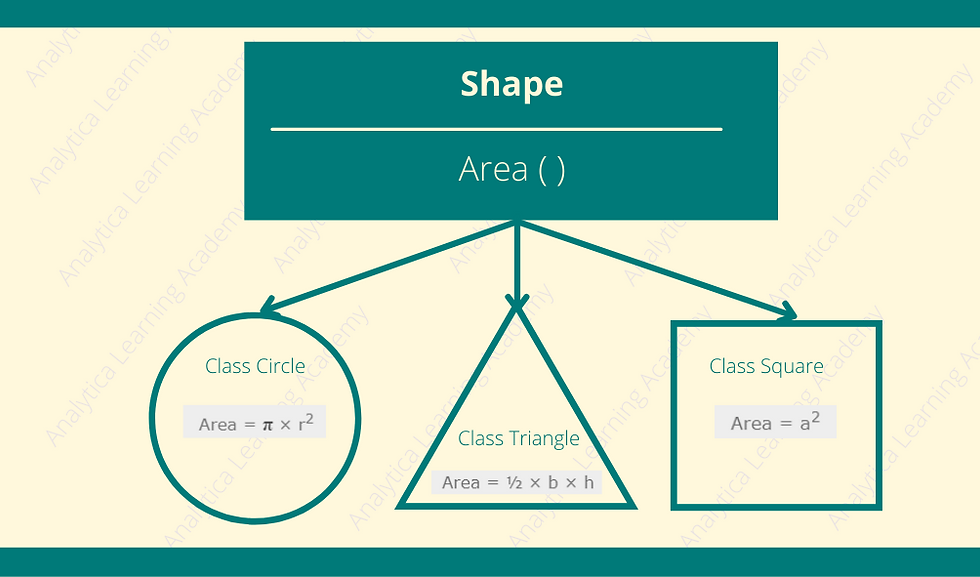
Pluggability goal of object-oriented programming is implemented in the polymorphism principle. Pluggability is also referred to as substitutability.
Comments