Object-Oriented Programming Core Concepts Part 1
- Rosmina Joy Cabauatan
- Apr 7, 2021
- 4 min read
Abstraction
Abstraction is one of the prime concepts used to simplify programming problems. It is a mechanism and practice to reduce and factor out details to focus on a few ideas at a time. In applying abstraction, a programmer hides all the relevant data about an object to reduce complexity and increase efficiency.
Abstraction is one of the fundamental concepts of object-oriented programming (OOP) languages. Its main goal is to handle complexity by hiding unnecessary details from the user. It enables the user to implement more complex logic on top of the provided abstraction without understanding or even thinking about the hidden complexity. The figure below shows how abstraction is implemented both as an interface or abstract class.
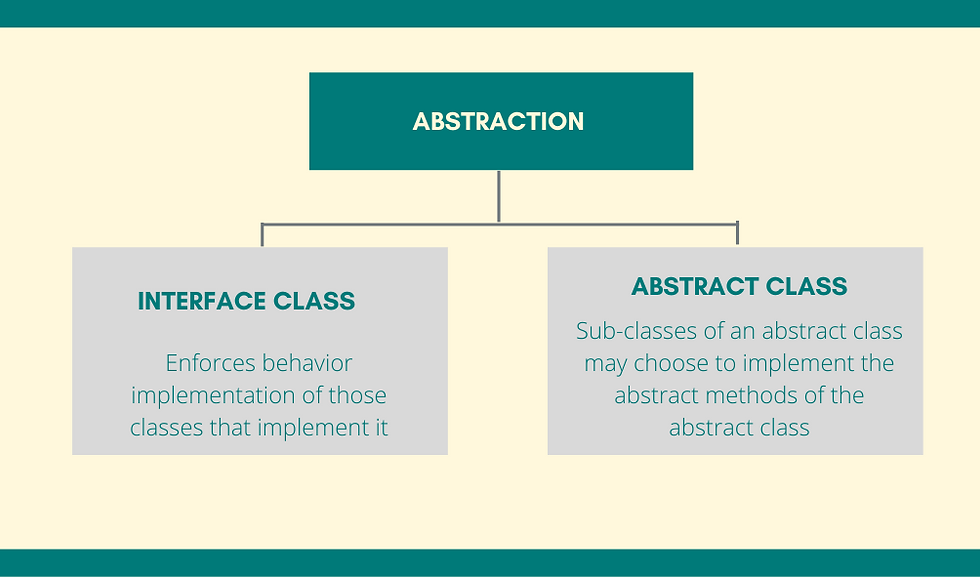
Structured Programming
There are several tasks at which an application needs to sequentially, selectively, and repeatedly perform. Structured Program Theorem allows these by sequential execution of subprograms, execution of one or more subprograms according to the validity of expression, and repeated execution of subprograms as long as an expression is valid. The figure below shows how structured programming is implemented in Java programming. The main program has several sub-functions or sometimes referred to as subprograms. One function calls another function in either a sequential or selective manner. Similar to other languages, this concept is applied in Java through control structures.

Procedural Programming
A characteristic of structured programming influenced the procedural programming approach based on the concept of calling a procedure. Procedures are routines, subroutines, or functions that comprise a series of steps to be carried out by a system. A procedure is executed at any point in the process of program execution.
Routines are grouped into functions in procedural programming. A function can call another function. You don't have to understand each line of code, just what each function does. You can also hide data to be accessible only within a function. Encapsulation is the term used for this concept in procedural programming.
Object-Oriented Programming (OOP)
Object-oriented programming takes encapsulation by localizing data, and associated operations into a mini-program called an object. An object-oriented program is an ecosystem of objects that interact with each other. Allan Hollub, a programmer, associates an object-oriented system as a bunch of intelligent animals as objects inside a machine, talking to each other by sending messages to one another.
Object-oriented programming is a model that is based upon the concept of objects. Objects contain data in the form of attributes and code in the way of methods. In OOP, programs are created using the idea of objects that interact with the real world. OOP languages are various, but the most popular ones are class-based, meaning that objects are instances of classes, which also determine their types.
OOP takes abstraction farthest by allowing you to group related data and operations into different types of objects. You create your data types, which are types of objects. Each of these data types is called objects. Creating your classes allows you to design a program so that it is intuitive to remember how it is organized. A good example is as follows:
Creating classes that represent real-world entities.
Creating classes to have specific responsibilities so that when you need to update a piece of code, you know exactly where to look for it
Java is one of the languages that apply object-oriented programming. The figure below shows how procedures are refined and how data as parameters are passed between procedures. The figure also shows how operations and attributes are encapsulated in different objects. These objects are classes that represent real-world entities with specific responsibilities. These responsibilities are performed through the operations of the objects.
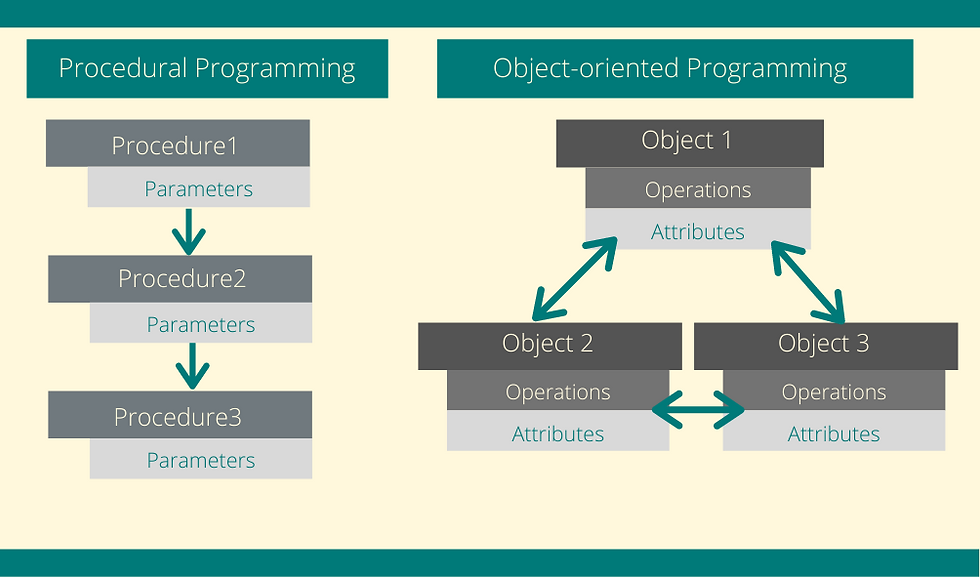
Goals of Object-Oriented Programming
Comprehensibility
Maintainability
Reusability
Pluggability
Comprehensibility can be achieved by making your code easy to understand. It should be easy for anyone to comprehend what a specific part of your does. If you need to fix or change something, it should be intuitive to know where the lines of code you need to change are located.
Maintainability can be achieved by making code easy to modify. Always remember that 80% of the cost of a program is in maintenance. This is because of new features that are added to a system and in fixing bugs. So, it should be easy to locate where to add new features or where problems exist.
Reusability can be achieved by making old codes as building blocks for new code. This is by creating an interchangeable component that can substitute for one another.
Pluggability is the essence of reusability. This goal can be achieved when a specific part of the code is instantiated which does not require a lot of changes before its implementation.
Class
An entity in an application is represented as a class in Java programming. A class is
a blueprint for objects to follow a specific schema defined in the class. A class defines the behavior for objects of its type;
a representation of a collection of properties (data and functions) for all its objects;
supports a template for creating objects which bind code and data;
acts as a means to define methods and data; and
helps in maintaining access specifications for member variables using access specifiers.
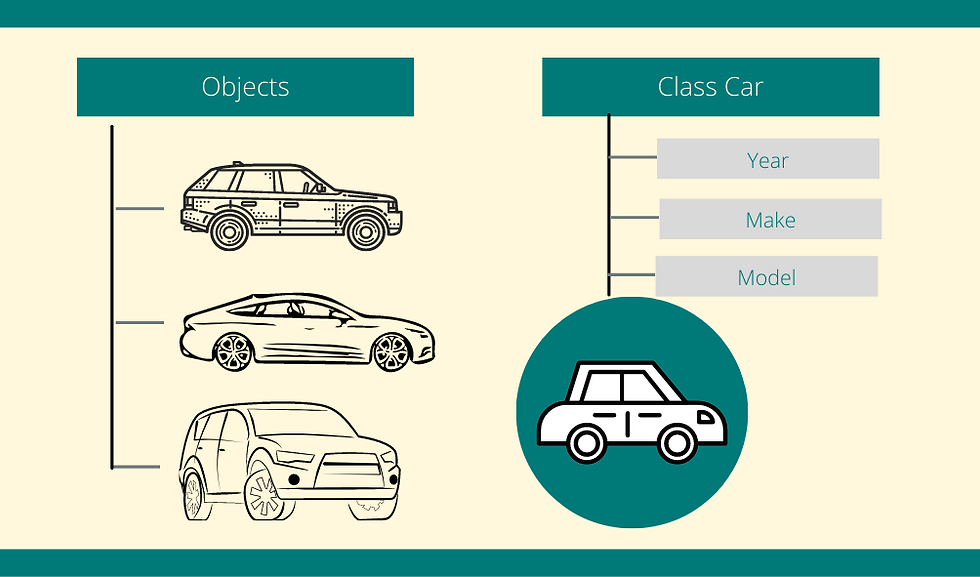
Objects
An object represents a real-life entity. It is composed of attributes, behavior, and has an identity. In Java programming, an object is derived from its class. The class has properties such as its state and methods. Identity is the name assigned to an object.
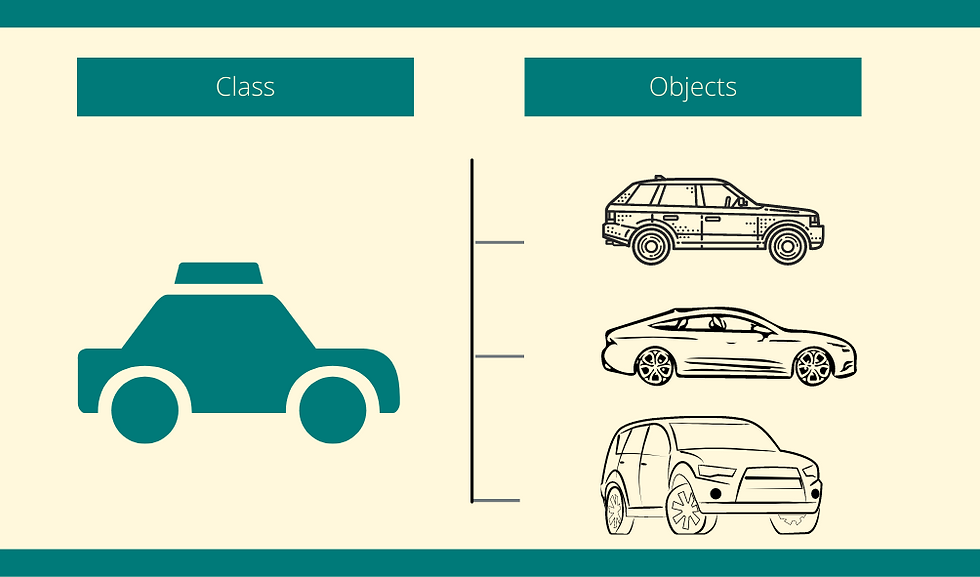
What is a Class and an Instance of a Class?
A class is the definition of an object. An instance is the created object of a class. For example, if your car class is 2018 BMW, a lot of instances of this car are available out there. However, there is only one instance of your car.
What is an object?
An object has attributes which are the properties and components of an object. An object also has methods which are behaviors or routines of the attributes. The following presents the attributes and methods of an object car. In programming, attributes are commonly referred to as variables and methods as functions.
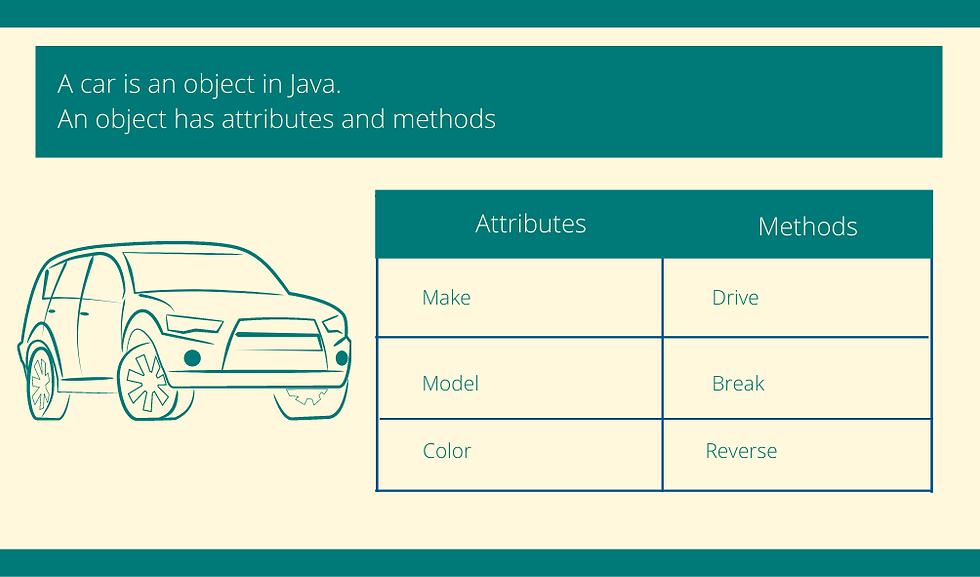
Commentaires